π» Week 01 Lab
Data Types in Python
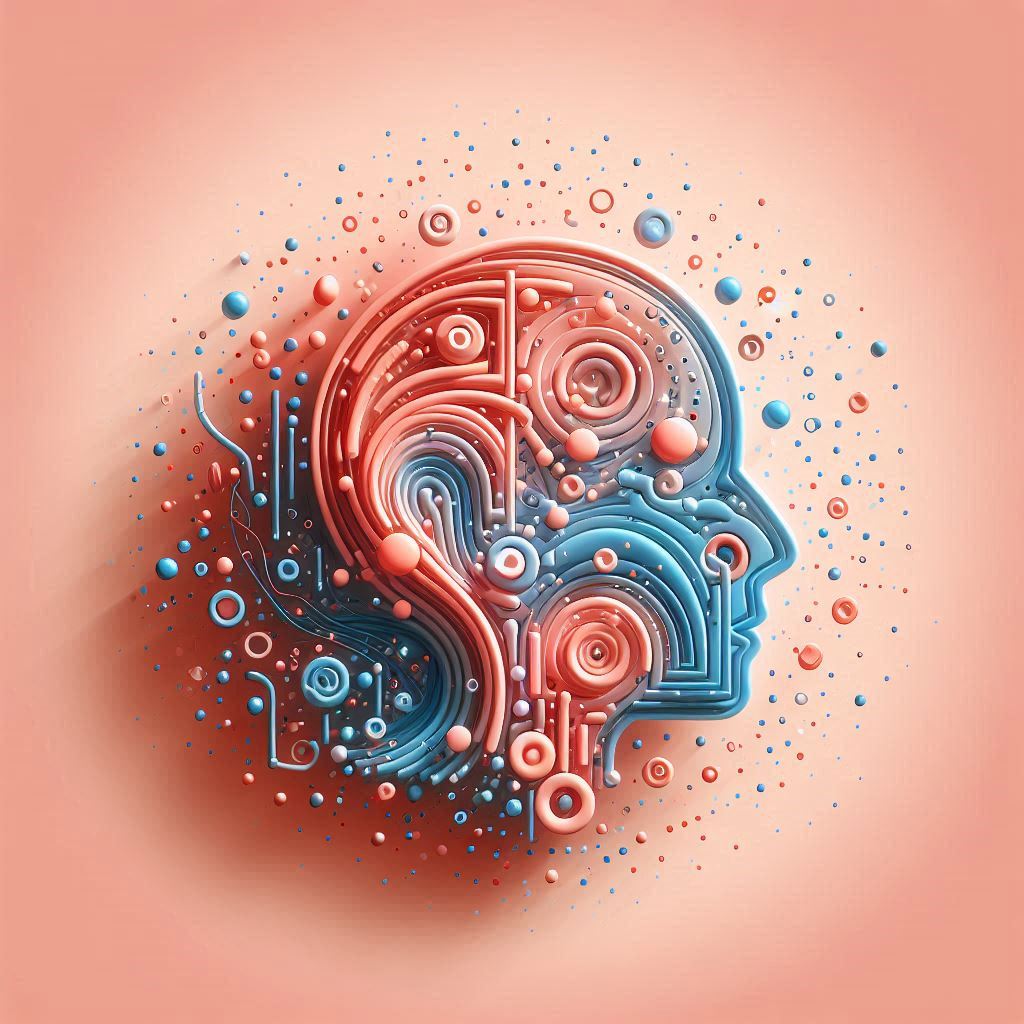
This is your first lab session! π
πLocation: Friday 4 October 2024, all day.
β οΈ Please check your timetable for the latest class locations. There have been changes because the original classrooms were too small for us.
Check your timetable for the precise time of your lab.
π₯ Learning Objectives
We have goals we want you to achieve by the end of this lab! By the end of this lab, you should be able to:
π Preparation
At DS105, we donβt think teaching is restricted to the classroom. We believe learning happens anytime, anywhere, and that frequent human-to-human communication is key to a successful learning experience.
The labs are the place where you get to practice what you have learned in the lectures and in the self-study material, secured in the knowledge that your instructors are there to help you if you get stuck.
You will feel better prepared for this first lab if you have completed this weekβs exercise.
If you came to the lab without doing the exercise, donβt worry! Follow along with your instructor and practice it after the lab.
π£οΈ Roadmap
Here is how we will achieve these goals:
π€ Part I: Introductions (15 min)
π§βπ« TEACHING MOMENT: Your chance to get to know your classmates and instructors.
Each instructor will have their own way of doing this, but you can expect to introduce yourself to the class and hear a bit about your instructorsβ backgrounds and interests.
π‘ TIP: Whenever you see a π§π»βπ« TEACHING MOMENT in the lab roadmap, it means your class teacher will be talking to you, and they deserve your full attention. Please do not work on the lab exercises during these moments. You will have plenty of time to do so later on.
π Choose your adventure (15 min)
What you will be doing in the next part of the lab depends on whether you have Python installed on your computer or not. Your class teacher will be going around the room to help you with this.
Q: How do you know if you have Python installed?
A: You can check by typing
python --version
in your terminal. If you see a version number, and it starts with the number 3, you have a valid Python installed.
Python Installed
If you have Python installed on your computer, you can skip to the next section.
π« Python Not Installed
If you do not have Python installed on your computer, the most productive use of your time will be to set up an account on our Nuvolos Cloud platform by following the instructions in the Setting up Nuvolos guide.
Do not worry if you canβt complete the sections below. You will have time to catch up later.
π‘ TIP: Whenever the class teacher asks for your attention, please pause your setup, listen to the instructions and take notes of what they are saying. This will help you catch up with the rest of the class once you have your Python environment ready.
π‘ TIP: After the lab, reserve some time to go over the π W01 Formative Exercise and complete it on Nuvolos. Post questions to the #help
channel on Slack or attend a support session (office hours or drop-in session) if you get stuck.
Part II: Enter the Python shell & its variables (30 min)
π‘ TIP: This part contains π― ACTION POINTS. Whenever you see these words in the lab roadmap, this means it is your time to shine! Work on the steps independently (or with a colleague near you). The class teacher will go around the room to help you if you need any assistance.
π― ACTION POINTS
Follow the steps below by yourself whilst paying extreme attention to the instructions. Keep notes of your observations and questions. Your class teacher will ask you about them later.
Open the terminal app on your computer. If you are unsure how to do this, ask your class teacher for help.
Type
python
and hit ENTER. You should see a prompt like this:3.x.xx (default, MON DAY YEAR, HH:MM:SS) Python -NUMBERS] on linux [GCC SOME"help", "copyright", "credits" or "license" for more information. Type >>>
Type
print("Hello, world!")
and hit ENTER. You should see the following output:! Hello, world
NOTE: When you type a command like
print()
inside the Python shell, it will execute the command and show you the output immediately. Theprint()
function is used to display whatever is inside the parentheses on the screen. In this case, you just printed the text βHello, world!β to the screen, nothing more.Type
exit()
and hit ENTER to exit the Python shell.If you feel like itβs time to take a break from Python, you can always type
exit()
to leave the Python shell and return to the terminal.Enter the Python shell again by typing
python
and hitting ENTER.Create your first variable. Type the following command:
= "Hello, world!" my_variable
This command creates a variable called
my_variable
and assigns, with the use of the=
operator the text βHello, world!β to it.What is the point of a variable? Well, variables are used to store data that you can use later in your program. At this point, our data is just some silly text, but later on, you will see how variables can store numbers, lists, and even more complex data structures.
Whatβs inside a variable? Type the following on the Python shell and hit ENTER:
my_variable
You should see the text βHello, world!β printed on the screen. This is the content that is stored inside the
my_variable
variable.π Think about it: This question might sound silly, but observe what you see and make your best-educated guess. What is the difference between typing
my_variable
+ ENTER andprint(my_variable)
+ ENTER? Write down your thoughts somewhere. Your teacher will ask about it later.What is the type of a variable? Type the following command and hit ENTER:
type(my_variable)
You should see the following output:
<class 'str'>
This output tells you that the variable
my_variable
is of typestr
, which stands for string. A string is a sequence of characters, like the text βHello, world!β.π Think about it: What do you think the
type()
function does? Write down your thoughts somewhere. Your teacher will ask about it later.Create a different type of variable. Type the following command and hit ENTER:
= 42 my_number
This command creates a variable called
my_number
and assigns the number42
to it.Then type the following command and hit ENTER:
type(my_number)
You should see the following output:
<class 'int'>
π Think about it: What do you think the
int
type represents? Write down your thoughts somewhere. Your teacher will ask about it later. Feel free to browse the Web or use an AI chatbot after you wrote down your notes, to check if your intuition matches the definition of the variable.Use Python as a calculator. Observe what happens after type the following command and hit ENTER:
+ 10 my_number
Update the value of a variable. Type the following command and hit ENTER:
= my_number + 10 my_number
Then type the following command and hit ENTER:
my_number
You should see the number
52
printed on the screen. This is the result of adding10
to the previous value of42
.Once again, what is the difference between typing
my_number
+ ENTER andprint(my_number)
+ ENTER? Write down your thoughts somewhere. Your teacher will ask about it later.How would you save a bunch of numbers in a variable? With a
list
, of course. Type the following command and hit ENTER:= [1, 2, 3, 4, 5] my_list
This command uses the square brackets
[]
to create a list of numbers and assigns it to the variablemy_list
.Then type the following command and hit ENTER:
my_list
You should see the list
[1, 2, 3, 4, 5]
printed on the screen.What is contained in the 3rd element of the list? Type the following command and hit ENTER:
2] my_list[
You should see the number
3
printed on the screen.π Think about it: Why did we use the number
2
to access the 3rd element of the list? Write down your thoughts somewhere. Your teacher will ask about it later.Drop duplicates with
set
. Type the following command and hit ENTER:= [1, 2, 3, 4, 5, 1, 2, 3, 4, 5] my_list
This command creates a list with duplicates. Then type the following command and hit ENTER:
my_list
You should see the list
[1, 2, 3, 4, 5, 1, 2, 3, 4, 5]
printed on the screen.How big is this list? The
len()
function can help you with that. Type the following command and hit ENTER:len(my_list)
You should see the number
10
printed on the screen. This is the length of the list.What if I donβt care about repetitions? You could convert this
list
to aset
to remove duplicates. Type the following command and hit ENTER:= set(my_list) my_set
Then type the following command and hit ENTER:
my_set
You should see the set
{1, 2, 3, 4, 5}
printed on the screen. Sets are similar to lists, but they do not allow duplicates.How big is this set? Type the following command and hit ENTER:
len(my_set)
You should see the number
5
printed on the screen. This is the length of the set.Different to lists, you cannot access elements in a set by their position. Sets are unordered collections of unique elements.
Now, meet the dictionary. Lists are very useful in data science, because very often we are dealing with a collection of data. But sometimes we need to store multiple lists, and it helps to give each one of them a meaningful name. This is where dictionaries come in. Type the following command and hit ENTER:
= {"name": "Alice", "age": 25, "city": "London"} my_dict
This command creates a variable called
my_dict
and assigns a dictionary to it. A dictionary is a collection of key-value pairs. In this case, the keys are"name"
,"age"
, and"city"
, and the values are"Alice"
,25
, and"London"
.Then type the following command and hit ENTER:
my_dict
You should see the dictionary
{'name': 'Alice', 'age': 25, 'city': 'London'}
printed on the screen.Use the keys (and square brackets) to access the values in the dictionary. Type the following command and hit ENTER:
"name"] my_dict[
You should see the text
"Alice"
printed on the screen.Mixing it up: dictionaries of lists. Say you have collected hourly temperature data about European capitals. You could chose to save each one of the temperature measurements in lists of 24 elements (to represent each hour of the day). But then you would have to create a list for each city, and remember which list corresponds to which city. This is where dictionaries of lists come in.
Say you have that data saved somewhere. You could manually create a dictionary of lists like this:
= { temperatures "London": [10, 11, 12, 13, 14, 15, 16, 17, 18, 19, 20, 21, 22, 23, 24, 25, 26, 27, 28, 29, 30, 31, 32, 33], "Paris": [9, 10, 11, 12, 13, 14, 15, 16, 17, 18, 19, 20, 21, 22, 23, 24, 25, 26, 27, 28, 29, 30, 31, 32], "Berlin": [8, 9, 10, 11, 12, 13, 14, 15, 16, 17, 18, 19, 20, 21, 22, 23, 24, 25, 26, 27, 28, 29, 30, 31] }
This command creates a variable called
temperatures
and assigns a dictionary of lists to it. The keys are the names of the cities, and the values are lists of temperatures per hour (from 00h to 23h).Then type the following command and hit ENTER:
temperatures
You should see the dictionary of lists printed on the screen.
Use the keys (and square brackets) to access the lists in the dictionary. Type the following command and hit ENTER:
"London"] temperatures[
You should see the list of just one of the cities printed on the screen.
Compare the temperatures of two cities. Type the following command and hit ENTER:
"London"] == temperatures["Paris"] temperatures[
You should see the output
False
printed on the screen. This is because the temperatures are different in each city.π Think about it: What do you think the
==
operator does? Write down your thoughts somewhere. Your teacher will ask about it later.π Hereβs a little challenge: Use the Python shell to check which city has the highest temperature at 12pm.
Part III: Your questions, answered (15 min)
This is a π£οΈ CLASSROOM DISCUSSION moment.
Your class teacher will ask about the answers you wrote down during the exercises above.
π‘ TIP: Whenever you see a π£οΈ CLASSROOM DISCUSSION moment in the lab roadmap, it means your class teacher will be asking questions to the whole class. Some class teachers might use a live Mentimeter poll, others might ask you to raise hands. Show your appreciation to them and interact as much as possible.
Part IV: Pythonβs data types (15 min)
π§βπ« TEACHING MOMENT
Before they give you a solution to step 18 above, your class teacher will guide you through the different types of data that Python can handle. They will explain the differences between primitive and non-primitive data types, and show you how you will eventually use them in your data science projects.
ποΈ Useful links
Now what?
You have completed the lab! π
If something was not clear during the lab, first of all know that programming is hard, and itβs okay to feel lost sometimes. We promise you that, eventually, everything will *click* and you will feel like a Python master. Keep practicing, and donβt be afraid to ask questions.
Got questions?
Post any question you might have to the #help
channel on Slack. On the first week, Jon will be checking Slack all day on Friday to help you with any questions you might have. Alternatively, attend a support session (office hours or drop-in session) early next week.
Want to learn more about Python?
Our colleagues at the LSE Digital Skills Lab are offering a series of workshops to help you get started with Python. Check out the links below:
- Python Pre-sessional workshops
- Or the simpler, self-paced Introduction to Python for Data Science - Dataquest course on Moodle (read the instructions carefully to learn how to access the Dataquest resource).
Want to practice more?
Then you are ready to face the π W02 Formative Exercise on Nuvolos. This exercise will help you consolidate what you have learned today.