📝 W02 Formative Exercise: Loops, Lists and Dictionaries
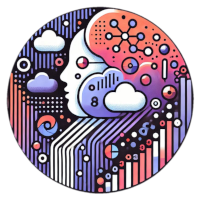
Briefing
⏳ | Duration | 1-2 hours depending on your familiarity with Python basics. |
📂 | Folder to Use in Nuvolos | Create a new notebook under the folder Week 02 - Python Collections & Loops/ |
📥 | Prerequisites | - Complete the 📝 W01 Formative from last week - Attempt the take-home exercise from the 💻 W01 Lab even if you don’t quite feel like have the necessary skills yet. |
Task 1: Take new DataQuest lessons
The activities under the 💻 W01 Lab required a good grasp of print()
statements, formatting of strings, and the notion of list
in Python. Some of this knowledge was covered in the DataQuest lessons we asked you to take last week, and some of it was delivered in class by your class teacher.
If you tried solving the take-home activity, even without learning about for
loops as I hope you did 1, you probably realised that the knowledge you acquired about lists is insufficient to solve it.
It’s therefore, time to fill in that gap.
🎯 ACTION POINTS:
Create a new Python notebook in your Nuvolos account under the folder “Week 02 - Python Collections & Loops”.
- Open VS Code in Nuvolos.
- Right-Click the folder “Week 02 - Python Collections & Loops” and select “New File”.
- Name the file “W02 - DataQuest Lessons.ipynb” (precisely as written here).
- Add a Markdown cell at the top of the notebook and type the title
# DataQuest Lessons - Week 02
.
Use this notebook to take notes on the DataQuest lessons you will be completing. Keep adding new Markdown cells as you progress through the lessons, and try to use Markdown to format your notes.
Dedicate some time to complete the DataQuest lessons below and add notes of what you learn to the notebook you just created.
Task 2: Understand the solution to the 🏡 Take-Home Activity
Now, with this new knowledge of for
loops in mind, let’s break down the solution into parts. Re-read the task instructions if you need to refresh your memory.
Either create a separate notebook to store these solutions or add them to the W01 Lab Notebook you used last week.
Step 1: Create the Data
The first thing we need to do is to create the data for the cities we want to compare. We decided to save everything into lists, so we can easily access the data later on.
# Relevant days of the week for the forecast
= ["Fri", "Sat", "Sun", "Mon", "Tue", "Wed", "Thu"]
days_of_week
# London Data
= [7, 9, 11, 10, 8, 6, 7]
london_forecast_max = [2, 3, 4, 3, 2, 1, 2]
london_forecast_min = ["🌧️", "⛅", "☀️", "🌧️", "⛅", "☁️", "🌧️"]
london_conditions
# Paris Data
= [8, 10, 12, 11, 9, 7, 8]
paris_forecast_max = [3, 4, 5, 4, 3, 2, 3]
paris_forecast_min = ["⛅", "⛅", "☀️", "☁️", "🌧️", "🌧️", "☀️"] paris_conditions
☝️ Go ahead and create a new Python cell in your Jupyter Notebook and paste the code above. Maybe add a Markdown cell above it to explain how we got this data (we just googled the weather forecast for the next week in London and Paris).
Step 2: Start small
We need to represent the min and max temperatures as well as the dominant weather condition for each day side by side for both cities.
Let’s ignore the other strings we need to print()
and focus on the lines where we need to use data from the lists. Essentially, each day must be represented as follows:
Fri: 🌧️ 7°C (2-7°C) | ⛅ 8°C (3-8°C)
Always start small!
Before you try to solve for all days, try to create the code that would work for just a single day, the first one in each list for example.
Remember (from your DataQuest lessons and W01 Lab instructor’s explanation):
Concept | Explanation |
---|---|
Accessing the first element of a list | list_name[0] |
Adding tabs to a string | "\t" |
Combining text and variables in a print() statement |
Using f-strings (e.g., f"Max temp: {max_temp}°C" ) |
Go even smaller!
Before you even print the line, think about the first part of the line, the day of the week. How would you print just that?
Create a new Python cell in your notebook and try to print the first day of the week:
# Print the day of the week
print(days_of_week[0] + ":")
Then, erase the line above (or keep it to help you review) and try writing it again using an f-string:
# Print the day of the week using an f-string
print(f"{days_of_week[0]}:")
Only once you had success with this, add some space:
# Print the day of the week using an f-string and adding a tab
print(f"{days_of_week[0]}:\t")
Start with just the first city
Then, let’s keep increasing the complexity. Try to print the first day of the week for London:
# Print the day of the week and the max and min temperatures for London
print(f"{days_of_week[0]}:\t{london_conditions[0]} {london_forecast_max[0]}°C ({london_forecast_min[0]}-{london_forecast_max[0]}°C)")
Add the second city
Finally, add the data for Paris:
# Print the day of the week and the max and min temperatures for London and Paris
print(f"{days_of_week[0]}:\t{london_conditions[0]} {london_forecast_max[0]}°C ({london_forecast_min[0]}-{london_forecast_max[0]}°C)\t|\t"
f"{paris_conditions[0]} {paris_forecast_max[0]}°C ({paris_forecast_min[0]}-{paris_forecast_max[0]}°C)")
Loop through all days
Now, you can try to loop through all days of the week and print the forecast for both cities side by side.
# Let's add the 'title'
print("""
WEEKLY FORECAST COMPARISON
=============================
\tLONDON\t\t\tPARIS\t\t\t
""")
# Loop through all days of the week
for i in range(len(days_of_week)):
# Print the day of the week and the max and min temperatures for London and Paris
print(f"{days_of_week[i]}:\t{london_conditions[i]} {london_forecast_max[i]}°C ({london_forecast_min[i]}-{london_forecast_max[i]}°C)\t|\t"
f"{paris_conditions[i]} {paris_forecast_max[i]}°C ({paris_forecast_min[i]}-{paris_forecast_max[i]}°C)")
Notice how the code inside the for
loop is essentually the same as the code you wrote for the first day. The only difference is that you are using the variable i
to access each respective element of the lists.
What is the role of range()
in the for
loop?
The range()
function generates a sequence of numbers that the for
loop can iterate over. In this case, we are generating a sequence of numbers from 0
to 6
(the length of the days_of_week
list).
Step 3: Make it neater
The code above works, it’s fine but the name of our variables are a bit long. Let’s create new variables to store the data for each day and make the code inside the loop a bit more readable.
# Let's add the 'title'
print("""
WEEKLY FORECAST COMPARISON
=============================
\tLONDON\t\t\tPARIS\t\t\t
""")
# Loop through all days of the week
for i in range(len(days_of_week)):
# Create new variables to store the data for each day
= f"{london_conditions[i]} {london_forecast_max[i]}°C ({london_forecast_min[i]}-{london_forecast_max[i]}°C)"
london_day = f"{paris_conditions[i]} {paris_forecast_max[i]}°C ({paris_forecast_min[i]}-{paris_forecast_max[i]}°C)"
paris_day
# Then here I can just use the new variables
print(f"{days_of_week[i]}:\t{london_day}\t|\t{paris_day}")
Once you have working code, it’s always a good idea to make it shorter. As a rule of thumb, try to write code that doesn’t go beyond 80 characters in a single line. This makes it easier to read and understand. (Our code is not there yet, but I’ll leave it as an exercise for you to make it shorter.)
Step 4: Calculate Weekly Statistics
The specifications asked for the average high and low temperatures for each city, as well as the temperature range.
The code below calculates these values for Paris and London:
# Calculate averages and ranges for Paris
= round(sum(paris_forecast_max) / len(paris_forecast_max),1)
paris_avg_high = round(sum(paris_forecast_min) / len(paris_forecast_min),1)
paris_avg_low = max(paris_forecast_max) - min(paris_forecast_min)
paris_temp_range
# Calculate averages and ranges for London
= round(sum(london_forecast_max) / len(london_forecast_max),1)
london_avg_high = round(sum(london_forecast_min) / len(london_forecast_min),1)
london_avg_low = max(london_forecast_max) - min(london_forecast_min) london_temp_range
Here, round()
is used to round the averages to one decimal place. The sum()
function is used to calculate the sum of all elements in a list, and len()
is used to calculate the length of a list.
Notice: even though we thought about this solution now, we will need this code to appear before the loop that prints the weekly forecast comparison. This is because we need to print the averages and ranges after the comparison. To make this work, you will need to move the code to a cell just below where you create the data for the cities.
Step 5: Count Rainy Days
The last part of the task was to count the number of rainy days for each city. This can be done by counting the number of occurrences of the rain emoji in the conditions list.
This is done by a a mix of a for
loop and an if
statement:
= 0
paris_rain_days for condition in range(len(paris_conditions)):
if paris_conditions[condition]=="🌧️":
+=1
paris_rain_days
= 0
london_rain_days for condition in range(len(london_forecast_conditions)):
if london_forecast_conditions[condition]=="🌧️":
= london_rain_days + 1 london_rain_days
Notice: This code should also be placed before the loop that prints the weekly forecast comparison.
Step 6: Print the Weekly Summary
Finally, we need to print the weekly summary. This is done by adding the following code after the loop that prints the weekly forecast comparison:
print(f"""
WEEKLY SUMMARY
=============================
\t\tLONDON\t\tPARIS\t\t
Avg High:\t{london_avg_high}°C\t|\t{paris_avg_high}°C
Avg Low:\t{london_avg_low}°C\t|\t{paris_avg_low}°C
Temp Range:\t{london_temp_range}°C\t|\t{paris_temp_range}°C
Rainy Days:\t{london_rain_days}\t|\t{paris_rain_days}
""")
This code uses f-strings to print the average high and low temperatures, the temperature range, and the number of rainy days for each city.
Step 7: Ask questions
Was there anything here that was confusing? Did you find a ‘bug’ in the code (something that should work but doesn’t)?
Before you proceed, write a question to the #help
channel on Slack!
Task 3: Take some new DataQuest lessons
It’s time to learn some new Python concepts. Those will be essential for the Week 02 Lecture and Lab.
🎯 ACTION POINTS:
Dedicate some time to complete the DataQuest lessons below and add notes of what you learn to the notebook you created in Task 1.
Footnotes
Trying to tackle a coding challenge even though you don’t have all the necessary knowledge is a great way to learn.↩︎