Using Git for Team Collaboration
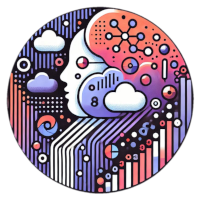
From Solo to Team Git
While you’ve been using Git for individual assignments, collaborating in a team requires new skills and workflows. This guide will help you transition from solo Git to team-based collaboration.
Essential Git Configuration for Team Work
Make sure your Git is configured properly to avoid common nagging issues:
Set your Git identity (if not already done)
It is important that the information you provide here matches exactly the information you have on your GitHub account.
git config --global user.name "Your Name" git config --global user.email "your.email@example.com"
📷 How to get your photo on GitHub commits
GitHub will use your GitHub profile photo on your commits. If you don’t have a photo, GitHub will search for your e-mail address on a website called Gravatar. If you have a Gravatar account and a photo associated with your email address, it will be used on your GitHub commits.
Configure pull strategy (⚠️ IMPORTANT for avoiding confusing messages)
git config --global pull.rebase false
Set your default branch name (most repos now use ‘main’ instead of ‘master’)
git config --global init.defaultBranch main
Set the default text editor for commit messages
git config --global core.editor "nano"
We’ve seen
nano
in 📝 W03 Formative. If you don’t set the above, you will get by defaultvim
as your text editor. People have been known to get stuck in a text editor for hours.
💡 Why pull.rebase false
is important
When you run git pull
in a team environment, Git can use two strategies to integrate changes:
- Merge (the default with
pull.rebase false
): Creates a merge commit when there are conflicts - Rebase: Rewrites history by placing your changes on top of the remote changes
For beginners, the merge strategy is safer and easier to understand. When not configured explicitly, Git will show a confusing message asking you to choose a strategy every time a conflict might occur.
Setting Up a Team Repository
🚨 In this course, you must use our GitHub Classroom repositories, so you won’t actually need to create the repository yourself.
We use GitHub Classroom to make it easier to manage the repositories for you and to mark your work.
Our Workflow Philosophy
In this course, we will use a common branching structure to manage the development of your project. This is an adapted version of the GitHub Flow workflow, a popular workflow for software development and collaboration.
You will be assessed on your ability to use this workflow.
Branch Naming Conventions
You can decide on your own branch naming conventions. These are just some suggestions
You can use GitHub’s default branch naming conventions. If your GitHub Issue is named, say, “#3 Test Feasibility of Google Maps API for our project”, your branch will be automatically named something like 3-test-feasibility-of-google-maps-api-for-our-project.
- I always tweak it a bit, like issue3-jonjoncardoso-test-google-maps-api.
You can use just the issue number and your username, like issue3-jonjoncardoso (but then it’s not easy to tell what the branch is about).
You can use your own naming conventions.
Git Collaboration Recipes
Here are practical recipes for common Git team workflows. Follow these step-by-step instructions like you would follow a cooking recipe.
🍳 Recipe: Starting Work on a New Task
Ingredients:
Things you must have sorted out before:
- There is a GitHub issue assigned to you
- (Optional) You have already created a dedicated branch via GitHub
Instructions:
If you want to create a new branch yourself, from the terminal
You need to check that your
main
branch is up to date first:git checkout main git pull
Then, create a new branch for your task:
git checkout -b issue42-username-short-description
Tell GitHub to track the new branch:
# origin is the name of the remote repository git push -u origin issue42-username-short-description
Go to the Issue page on GitHub and associate the branch with the issue.
Keep pushing and committing your work as you normally do.
If you have already created the branch on GitHub
Go to the Issue page on GitHub and associate the branch with the issue.
Copy the branch name from GitHub.
Create a new branch on your local machine:
# This will ensure your local repository # is up to date with the remote repository git fetch --all # Create a new branch on your local machine git pull origin issue42-username-short-description
Keep pushing and committing your work as you normally do.
🍲 Recipe: Keeping Your Branch Updated with Main
Ingredients:
- You’re already working on a feature branch
- Other team members have made changes to
main
Instructions:
How to update your branch with the latest changes from main
First, commit any changes you’ve been working on:
git add . git commit -m "Save current work in progress"
Change to the local
main
branch and pull the latest changes:git checkout main git pull
Switch back to your task branch and merge in the updates:
git checkout your-branch-name git merge main
If you encounter conflicts, resolve them as described in the Conflict Resolution Recipe.
Continue working on your updated branch.
🥗 Recipe: Creating a Pull Request
Ingredients:
Things you must have sorted out before:
- You’ve completed your work and pushed all changes to GitHub
- Your code is tested and ready for review
Instructions:
How to create a pull request on GitHub
Go to your repository on GitHub.
Navigate to the “Pull requests” tab near the top of the repository page.
Click the green “New pull request” button.
Set up your pull request:
- “base” dropdown: select
main
(this is where your changes will go) - “compare” dropdown: select your branch name
- “base” dropdown: select
Review the changes shown to make sure they’re what you expect.
Click the green “Create pull request” button.
Fill in the details:
- Title: Brief but descriptive summary of your changes
- Description: Explain what you did, why, and any testing you performed
- Link to related issues: Type “Closes #42” or “Relates to #42” to link to an issue
- Add any other team members as reviewers using the “Reviewers” section on the right
Click “Create pull request” to finalize it.
Notify your team that your code is ready for review.
🍱 Recipe: Reviewing a Teammate’s Pull Request
Ingredients:
Things you must have sorted out before: - You’ve been assigned to review a pull request - You understand the context of the changes
Instructions:
How to properly review code on GitHub
Open the pull request on GitHub.
Read the description to understand what changes were made and why.
Click on the “Files changed” tab to see the actual code modifications.
Review the code carefully:
- Does it solve the problem described in the issue?
- Is the code clear and well-documented?
- Are there any bugs or edge cases not handled?
- Does it follow the team’s coding standards?
Add comments on specific lines by hovering over the line number and clicking the blue “+” button that appears.
When you’re ready to submit your review:
- Click the “Review changes” button at the top right of the Files changed tab
- If everything looks good, select “Approve”
- If changes are needed, select “Request changes” and explain what needs to be fixed
- If you just want to provide feedback without approving/rejecting, select “Comment”
- Write any summary comments
- Click “Submit review”
Follow up with the author in person or via chat if you have questions or suggestions that are better discussed interactively.
🍜 Recipe: Resolving Merge Conflicts
Ingredients:
Things you must have sorted out before:
- Git has reported conflicts during a merge or pull
- You understand which files have conflicts
Instructions:
(Simpler) Using VSCode to resolve conflicts
Do the merge in the terminal as usual:
# Grab changes from main to your task branch git merge main
Go to the “Source Control” tab in VSCode.
Click on the files that are in conflict.
Open VS Code’s merge tool to see the changes and decide which version to keep.
Save the file and close the merge tool.
Stage the resolved file:
git add file-name
Commit the resolved file:
git commit -m "Resolve merge conflicts with main branch"
Push the resolved file to GitHub:
git push
How to resolve conflicts step-by-step by hand
When Git reports a conflict, first identify which files are affected:
git status
Open each conflicted file in your code editor. You’ll see sections marked with conflict markers:
<<<<<<< HEAD This is the code from the branch you're merging into (usually your current branch) ======= This is the code from the branch you're merging from >>>>>>> branch-name
Edit each file to resolve the conflict:
- Decide which version to keep, or combine them if appropriate
- Remove ALL conflict markers (
<<<<<<<
,=======
,>>>>>>>
) - Make sure the final code is correct and will run properly
After editing, mark each file as resolved:
git add path/to/resolved/file.py
Repeat steps 2-4 for each conflicted file.
Once all conflicts are resolved, complete the merge:
git commit -m "Resolve merge conflicts with main branch"
Continue with your work or push the changes to GitHub:
git push
🔮 Recipe: Handling Emergencies
Ingredients:
Things you must have sorted out before:
- You’re facing an urgent issue that needs immediate attention
- Your teammates have made changes you need
Instructions:
How to handle emergency situations
If someone says “I can’t push because you haven’t pulled my changes,” first save your work:
git add . git commit -m "Save work in progress"
Pull in their changes:
git pull
If you get conflicts, resolve them following the Conflict Resolution recipe.
If you encounter the message “Please specify how to reconcile divergent branches”:
git config pull.rebase false git pull
If you’ve made a mistake and need to undo your last commit (but haven’t pushed):
git reset --soft HEAD~1
If you’ve pushed a bad commit and need to undo it (use with caution!):
git revert HEAD git push